2022/10/10
pythonで作成したCUIでポップアップやファイルダイアログを使う
pythonでCUIアプリ(Character User Interface)を作成したときに、エラーなどのポップアップ(メッセージボックス)や、ファイル選択のダイアログを出す方法をまとめました。
使用するライブラリ
pythonでポップアップを出すライブラリはいくつかありますが、本ページでは、デフォルトでpythonに含まれている"tkinter"を使用します。
tkinter
Tcl/Tk の Python インターフェース
ポップアップ(メッセージボックス)
以下のプログラムでポップアップを実行します。
import tkinter
from tkinter import messagebox
tk = tkinter
tk.Tk().withdraw()
tk.messagebox.showinfo('showinfo', 'information')
tk.messagebox.showwarning('showwarning', 'warning')
tk.messagebox.showerror('showerror', 'error')
tk.messagebox.askquestion('askquestion', 'Yes or No ?')
tk.messagebox.askokcancel('askokcancel', 'OK or Cancel ?')
tk.messagebox.askretrycancel('askretrycancel', 'Retry or Cancel ?')
tk.messagebox.askyesno('askyesno', 'Yes or No ?')
tk.messagebox.askyesnocancel('askyesnocancel', 'Yes or No or Cancel ?')
TK().withdraw()
- tkinterを実行するとウインドウが自動で表示されるのですが、
CUIアプリではウインドウを使わないので、この関数でウインドウを消しています。
messagebox
- 定型のメッセージボックスをポップアップします。
ポップアップで押すボタンによって戻り値が異なります。
詳細は以下で説明していきます。
1. showinfo
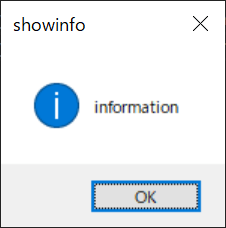
messagebox.showinfo('showinfo', 'information')
戻り値:"ok"
2. showwarning
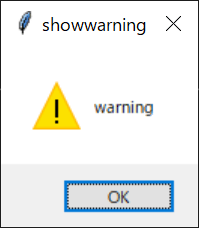
messagebox.showwarning('showwarning', 'warning')
戻り値:"ok"
3. showerror
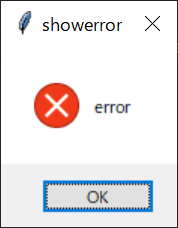
messagebox.showerror('showerror', 'error')
戻り値:"ok"
4. askquestion
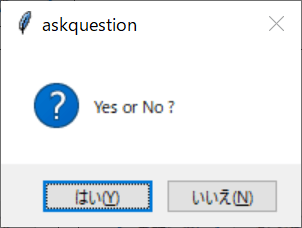
messagebox.askquestion('askquestion', 'yes or no ?')
戻り値:
はい(Y) ⇒ "yes"
いいえ(N) ⇒ "no"
5. askokcancel
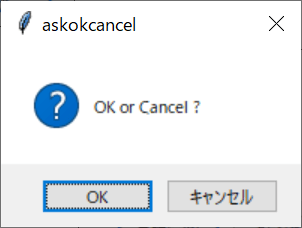
messagebox.askokcancel('askokcancel', 'OK or cancel ?')
戻り値:
OK ⇒ True
キャンセル ⇒ False
6. askretrycancel
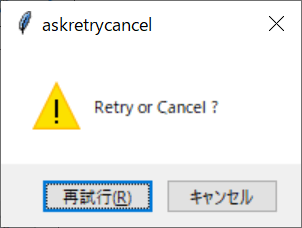
messagebox.askretrycancel('askretrycancel', 'Retry or Cancel ?')
戻り値:
再試行(R) ⇒ True
キャンセル ⇒ False
7. askyesno
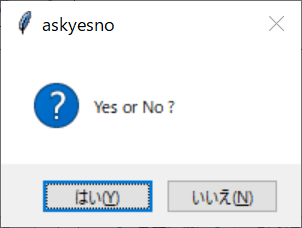
messagebox.askyesno('askyesno', 'Yes or No ?')
戻り値:
はい(Y) ⇒ True
いいえ(N) ⇒ False
8. askyesnocancel
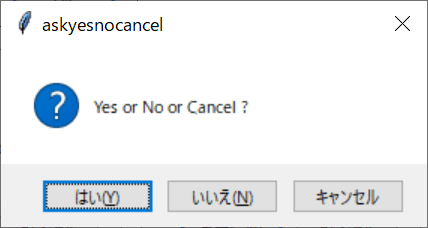
messagebox.askyesnocancel('askyesnocancel', 'Yes or No or Cancel ?')
戻り値:
はい(Y) ⇒ True
いいえ(N) ⇒ False
キャンセル ⇒ None
ファイル選択ダイアログ
以下のプログラムでダイアログを実行します。
import tkinter
from tkinter import filedialog
tk = tkinter
tk.Tk().withdraw()
type = [('テキストファイル','*.txt')]
path = tk.filedialog.askopenfilename(filetypes = type) #単一選択のみ
path = tk.filedialog.askopenfilenames(filetypes = type) #複数選択可
pathdirectory = tk.filedialog.askdirectory()
filedialog.askopenfilename
- ファイル選択ダイアログを開き、選択したファイルの絶対パスを戻します。
- filetypes:選択するファイル形式を指定できます。
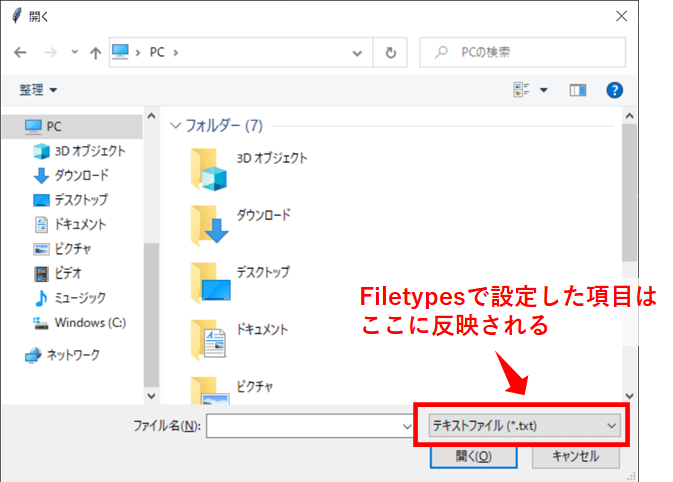
filedialog.askdirectory
- ファイル選択ダイアログを開き、選択したフォルダの絶対パスを戻します。
以上です。